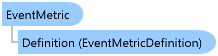
An EventMetric instance can only be created for a specific EventMetricDefinition which has been previously registered.
To protect against duplicates with the same instance name, EventMetric does not have public constructors. Instead, use the static EventMetric.Register method with appropriate arguments to identify the specific event metric definition under which to create an event metric instance with a designated instance name.
Once created, you can record samples for an event metric by:
Alternatively, for event metrics defined via attributes (see EventMetricAttribute and EventMetricValueAttibute), the event metric can be sampled directly from a user data object of the corresponding type which contained the attributes by simply calling WriteSample from the event metric definition or from the specific event metric instance. In this case all data is read and then written to the session file automatically.
For more information on how to take advantage of Event Metrics, see Developer's Reference - Metrics - Designing Event Metrics.
Sampled Metrics
An alternative to Event Metrics are Sampled Metrics. Sampled Metrics are designed to record a single, summarized value on a periodic basis. For more information on the difference between Sampled and Event Metrics, see Developer's Reference - Metrics - Sampled and Event Metrics. For more information on Sampled Metrics, see SampledMetric Class.
Viewing Metrics
Metrics are visible in the Session Viewer of the Gibraltar Analyst. Metrics are not displayed in the Loupe Live Viewer.
System.Object
Gibraltar.Agent.Metrics.EventMetric
Target Platforms: Windows 7, Windows Vista SP1 or later, Windows XP SP3, Windows Server 2008 (Server Core not supported), Windows Server 2008 R2 (Server Core supported with SP1 or later), Windows Server 2003 SP2
EventMetric Members
Gibraltar.Agent.Metrics Namespace
EventMetricSample Class
EventMetricDefinition Class
EventMetricAttribute Class
EventMetricValueAttribute Class
SampledMetric Class
Metrics - Introduction
Metrics - Sampled and Event Metrics