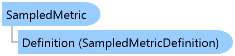
'Declaration
Public NotInheritable Class SampledMetric
public sealed class SampledMetric
Sampled Metrics are designed to record a single, summarized value on a periodic basis. Whenever a sample is recorded, the value is presumed to remain constant until the next sample is recorded. This works best when the data for each individual event is either unavailable or would be to inefficient to collect. To do this, the application has to do some degree of calculation or aggregation on its own and the useful trends have to be determined during development.
Windows Performance Counters are collected as Sampled metrics. Processor Utilization represents a good example of a sampled metric - It would be infeasible to record each time the processor was used for a task, and the underlying data isn't available.
For more information on how to take advantage of Sampled Metrics, see Developer's Reference - Metrics - Designing Sampled Metrics.
Event Metrics
An alternative to Sampled Metrics are Event Metrics. These offer more analysis options and can be much easier to record, particularly in multithreaded or stateless scenarios. For more information on the difference between Sampled and Event Metrics, see Developer's Reference - Metrics - Sampled and Event Metrics. For more information on Event Metrics, see EventMetric Class.
Viewing Metrics
Metrics are visible in the Session Viewer of Loupe Desktop. Metrics are not displayed in the Loupe Live Viewer.
SampledMetricDefinition pageMetricDefinition; //since sampled metrics have only one value per metric, we have to create multiple metrics (one for every value) if (SampledMetricDefinition.TryGetValue("GibraltarSample", "Database.Engine", "Cache Pages", out pageMetricDefinition) == false) { //doesn't exist yet - add it in all of its glory. This call is MT safe - we get back the object in cache even if registered on another thread. pageMetricDefinition = SampledMetricDefinition.Register("GibraltarSample", "Database.Engine", "cachePages", SamplingType.RawCount, "Pages", "Cache Pages", "The number of pages in the cache"); } //now that we know we have the definitions, make sure we've defined the metric instances. SampledMetric pageMetric = SampledMetric.Register(pageMetricDefinition, null); //now go ahead and write those samples.... pageMetric.WriteSample(pagesLoaded);
//Alternately, it can be done in a single line of code each, although somewhat less readable. //Note the WriteSample call after the Register call. SampledMetric.Register("GibraltarSample", "Database.Engine", "cachePages", SamplingType.RawCount, "Pages", "Cache Pages", "The number of pages in the cache", null).WriteSample(pagesLoaded);
//by using an object with the appropriate attributes we can do it in //one line - even though it writes multiple values. SampledMetric.Write(new CacheSampledMetric(pagesLoaded)); //To use the above line, you have to define the following class: /// <summary> /// Log sampled metrics using a single object /// </summary> [SampledMetric("GibraltarSample", "Database.Engine")] public class CacheSampledMetric { public CacheSampledMetric(int pagesLoaded) { Pages = pagesLoaded; Size = pagesLoaded * 8192; } [SampledMetricValue("pages", SamplingType.RawCount, "Pages", Caption = "Pages in Cache", Description = "Total number of pages in cache")] public int Pages { get; private set; } [SampledMetricValue("size", SamplingType.RawCount, "Bytes", Caption = "Cache Size", Description = "Total number of bytes used by pages in cache")] public int Size { get; private set; } }
System.Object
Gibraltar.Agent.Metrics.SampledMetric
Target Platforms: Windows 7, Windows Vista SP1 or later, Windows XP SP3, Windows Server 2008 (Server Core not supported), Windows Server 2008 R2 (Server Core supported with SP1 or later), Windows Server 2003 SP2
SampledMetric Members
Gibraltar.Agent.Metrics Namespace
Metrics - Designing Sampled Metrics
Metrics - Sampled and Event Metrics